Researchers of the Swiss Federal Institute of Technology in Zurich (ETH Zurich) built a state-of-the-art age and gender prediction models. Besides, they adopted the open source culture and shared the model structure and pre-trained weights of the production model for Caffe framework. So, we will mention how to run pre-built age and gender Caffe model within OpenCV in Python in this post.

Vlog
You can either follow this blog post or watch the following video to make age and gender predictions with deep learning within OpenCV in Python.
πββοΈ You may consider to enroll my top-rated machine learning course on Udemy
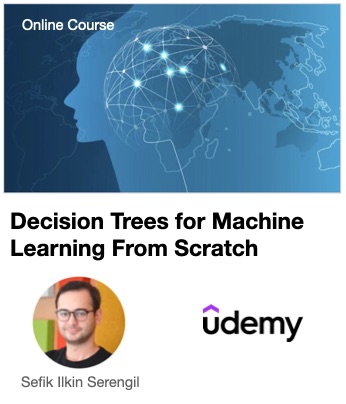
DeepFace library for python covers age prediction as well. You can run age estimation with a few lines of code.
Previous study
I couldn’t convert Caffe weights to Keras / TensorFlow pair before and I decide to re-train the age and gender models from scratch based on the published paper. Here, you can find my age and gender prediction models.
The original study was built on regular VGG model but I adopted VGG-Face as a base model. Even though they have both same structure, regular VGG trained on imagenet data set whereas VGG-Face trained on face data set. In other words, VGG-Face comes with an advantage to recognize some facial patterns. Because this study is mainly based on transfer learning and we will freeze early layers and we will not update weights of those locked ones. Training will be handled just in final fully connected layers.
Loading pre-built models
We can use the built model structure and pre-trained weights for Caffe framework within OpenCV. Besides, you don’t have to have Caffe framework in your environment. Here, you can find required files. I pasted the exact URLs of requires files as comments below. Then, we can load pre-built Caffe models within OpenCV.
#age model #model structure: https://data.vision.ee.ethz.ch/cvl/rrothe/imdb-wiki/static/age.prototxt #pre-trained weights: https://data.vision.ee.ethz.ch/cvl/rrothe/imdb-wiki/static/dex_chalearn_iccv2015.caffemodel age_model = cv2.dnn.readNetFromCaffe("age.prototxt", "dex_chalearn_iccv2015.caffemodel") #gender model #model structure: https://data.vision.ee.ethz.ch/cvl/rrothe/imdb-wiki/static/gender.prototxt #pre-trained weights: https://data.vision.ee.ethz.ch/cvl/rrothe/imdb-wiki/static/gender.caffemodel gender_model = cv2.dnn.readNetFromCaffe("gender.prototxt", "gender.caffemodel")
Face detection
OpenCV haar cascade is the easiest way to detect faces. Alternatively, some overperforming deep learning based approaches exist.
img = cv2.imread("img.jpg") haar_detector = cv2.CascadeClassifier("haarcascade_frontalface_default.xml"") def detect_faces(img): gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) faces = haar_detector.detectMultiScale(gray, 1.3, 5) return faces faces = detect_faces(img) for x, y, w, h in faces: detected_face = img[int(y):int(y+h), int(x):int(x+w)]
Pre-procesing
As I mentioned before, age and gender prediction models are based on VGG and the model expects 224×224 shaped inputs.
You can confirm the expected input shape in the model structure files having prototxt extensions. I copied the 1st 5 rows of the prototxt file below. This means that Caffe model expexts (1, 3, 224, 224) shaped inputs.
input = "data" input_dim = 1 input_dim = 3 input_dim = 224 input_dim = 224
Caffe model expects (1, 3, 224, 224) shaped inputs but OpenCV loads (224, 224, 3) shaped images even if we resize. blobFromImage function under deep neural networks module of OpenCV transforms read images to the expected shape.
detected_face = cv2.resize(detected_face, (224, 224)) #img shape is (224, 224, 3) now img_blob = cv2.dnn.blobFromImage(detected_face ) # img_blob shape is (1, 3, 224, 224)
Predictions
We can feed pre-processed input images to the built model and make predictions. OpenCV requires to set input and call forward commands respectively to make predictions.
age_model.setInput(img_blob) age_dist = age_model.forward()[0] gender_model.setInput(img_blob) gender_class = gender_model.forward()[0]
Firslty, age model returns 101 dimensional vector. In the original study, element wise multiplication is applied to the output vector and index vector.
output_indexes = np.array([i for i in range(0, 101)]) apparent_predictions = round(np.sum(age_dist * output_indexes), 2)
In addition, Gender model returns 2 dimensional model. If the 1st dimension value is greater than the 2nd one, then it is a woman. Vice versa, if the 2nd dimension value is greater than the 1st one, then it it is a man.
gender = 'Woman ' if np.argmax(gender_class) == 0 else 'Man'
Testings
You can see the testing results of pre-built age and gender models on game of thrones casting. We can obviously monitor how they grew up in years from season 1 to 8.
Age and gender in deepface
What’s more, deepface package for python offers age and gender prediction with a few lines of code.
#!pip install deepface from deepface import DeepFace demography = DeepFace.analyze("img4.jpg", actions = ['age', 'gender']) print("Age: ", demography["age"]) print("Gender: ", demography["gender"])
Furthermore, you can watch a demo for age and gender prediction in python with deepface.
Besides, deepface can access your webcam and run real time age and gender analysis as well.
Real time implementation
We can run age and gender prediction in real time. Here, you can find the source code of this task.
Conclusion
So, we’ve mentioned how to run pre-built Caffe models for age and gender prediction within OpenCV in Python. This one is built on regular VGG whereas its keras implementation is built on VGG-Face. My experiments show that VGG-Face based one overperforms than regular VGG based one.
Finally, I pushed the source code of this study to GitHub as a notebook. You can support this study if you starβοΈ the repo.
Like this blog? Support me on Patreon
Loading the caffe files fails:
age_model = cv2.dnn.readNetFromCaffe(‘age.prototxt’, ‘dex_chalearn_iccv2015.caffemodel’)
[libprotobuf ERROR /private/var/folders/nz/vv4_9tw56nv9k3tkvyszvwg80000gn/T/pip-req-build-ucld1hvm/opencv/3rdparty/protobuf/src/google/protobuf/text_format.cc:292] Error parsing text-format opencv_caffe.NetParameter: 1:1: Expected identifier, got: {
Traceback (most recent call last):
It seems that the content of prototxt or caffemodel is broken