Recently, Mish activation function is announced in deep learning world. Researchers report that it overperforms than both regular ReLU and Swish. The function is actually combination of popular activation functions.

It is a combination of identity, hyperbolic tangent and softplus. We should remember tanh and softplus functions at this point.
🙋♂️ You may consider to enroll my top-rated machine learning course on Udemy
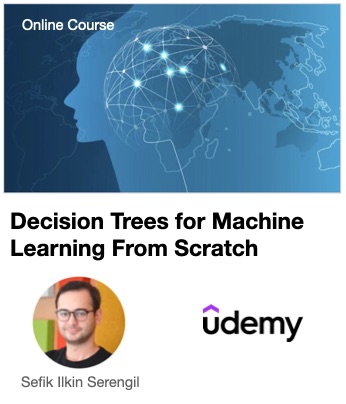
y = x.tanh(softplus(x))
whereas tanh(x) = (ex – e-x) / (ex + e–x) and softplus(x) = ln(1 + ex)
Combining these two functions shows the single mish function
mish(x) = x . (eln(1 + ex) – e-ln(1 + ex)) / (eln(1 + ex) + e-ln(1 + ex))
This becomes a very complex function but its graph will recall you Swish activation function.

Zoomed version of mish and swish shows how different these functions are. I draw these graphs with Desmos.

Implementing mish in python is an easy task.
def tanh(x): return (np.exp(x) - np.exp(-x))/(np.exp(x) + np.exp(-x)) def softplus(x): return np.log(1 + np.exp(x)) def misc(x): return x * tanh(softplus(x))
Derivative
We needed the mish function in feed forward step in neural networks. We will also need its derivative in backpropagation step.
y = x . (eln(1 + e^x) – e-ln(1 + e^x)) / (eln(1 + e^x) + e-ln(1 + e^x))
Firstly, we can simplify the misc function. This makes derivative calculation easy. Remember that e to ln(x) was x. In this case, e to ln(1+e^x) will be (1 + e^x)
y = x.(ex + 1 – 1/(ex + 1)) / (ex + 1 + 1/(ex+1)) = [x.((ex + 1)2 – 1)/(ex + 1)]/[((ex + 1)2 + 1)/(ex + 1)]
The both dividend and divisor have (ex + 1) as a divisor. We can simplify (ex + 1) terms.
y = [x.((ex + 1)2 – 1)]/[((ex + 1)2 + 1)]
Remember quotient rule. y’ = (f/g)’ = (f’.g – f.g’) / g2 . So, we can apply quotient rule to the term above.
f = [x.((ex + 1)2 – 1)] and g = [((ex + 1)2 + 1)]
Let’s find the derivative of f first
f’ = [x.((ex + 1)2 – 1)]’
Remember product rule. (a.b)’ = a’.b + a.b’. So, f is the multiplication of x and ((ex + 1)2 – 1).
f’ = x’ . ((ex + 1)2 – 1) + x. ((ex + 1)2 – 1)’
Finding the derivative of f requires to find [(ex + 1)2]
[(ex + 1)2]’ = 2.(ex + 1).(ex)’ = 2.(ex + 1).(ex)
Now, we can find the derivative of f
f’ = ((ex + 1)2 – 1) + x . (2ex(ex + 1)) = (ex + 1)2 – 1 + 2xex(ex + 1)
It is time to find the derivative of g
g’ = [((ex + 1)2 + 1)]’
We’ve already found the derivative of [(ex + 1)2]
g’ = 2ex.(ex + 1)
y’ = (f’.g – f.g’) / g2 = ( [(ex + 1)2 – 1 + 2xex(ex + 1)].[((ex + 1)2 + 1)] – [x.((ex + 1)2 – 1)][2ex.(ex + 1)] ) / ((ex + 1)2 + 1)2
Let’s say the term in the divisor to δ = ((ex + 1)2 + 1)
y’ = ( [e2x + 1 + 2ex – 1 + 2xe2x + 2xex][e2x + 1 + 2ex + 1] – [e2x + 1 + 2ex -1][2xe2x + 2xex] )/δ2
y’ = ( [e2x + 2ex + 2xe2x + 2xex][e2x + 2ex + 2] – [e2x + 2ex][2xe2x + 2xex] )/δ2
y’ = ([e4x +2e3x + 2e2x + 2e3x +4e2x + 4ex + 2xe4x + 4xe3x + 4xe2x + 2xe3x + 4xe2x + 4xex] – [2xe4x + 2xe3x + 4xe3x + 4xe2x] )/δ2
y’ = [e4x (1 + 2x – 2x) + e3x (2 + 2 + 4x + 2x – 2x – 4x) + e2x (2 + 4 + 4x + 4x – 4x) +ex (4 + 4x)]/δ2
y’ = [e4x + e3x (4) + e2x (6 + 4x) +ex (4 + 4x)]/δ2
y’ = ex . [e3x + e2x (4) + ex (6 + 4x) + 4(1 + x)]/δ2
Let’s set the term in the square brackets to ω.
This is the final form of the derivative of Mish activation function.
dy/dx = ex . ω / δ2
whereas ω = e3x + e2x (4) + ex (6 + 4x) + 4(1 + x) and δ = (ex + 1)2 + 1
Implementing the derivative of mish in python is a little costly.
def mish_derivative(x): omega = np.exp(3*x) + 4*np.exp(2*x) + (6+4*x)*np.exp(x) + 4*(1 + x) delta = 1 + pow((np.exp(x) + 1), 2) derivative = np.exp(x) * omega / pow(delta, 2) return derivative
Keras
We would mostly not implement neural networks architecture including feed forward and backpropagation steps from scratch. Building neural networks in high level APIs such as Keras is more common. We can adapt Mish into Keras even though it is not an out-of-the-box function.
def mish(x): return x * keras.backend.tanh(keras.backend.softplus(x)) """ model = Sequential() ... model.add(Conv2D(64,(3, 3), activation = mish)) ... """
Conclusion
So, we’ve mentioned a novel activation function Mish consisting of popular activation functions including identity, hyperbolic tangent tanh and softplus. Original paper skipped the derivative calculation step and gave the derivative directly. In this post, we’ve focused on the derivative calculation step also.
Even though, tens of functions has proposed so far, a few of them survived in daily studies. It seems that we would see several novel activation functions in the following days in deep learning world.
Let’s dance
These are the dance moves of the most common activation functions in deep learning. Ensure to turn the volume up 🙂
Like this blog? Support me on Patreon