We can do magnificent things with TensorFlow such as face recognition, facial expression recognition and computer vision. However, its main intent was research purpose. We can prototype a neural networks model easily and fast in this framework. Moving a prototype to production has an important role as well. Herein, we can use TensorFlow.js in production easily. Moreover, we can run tensorflow code either client side with traditional JavaScript or server side with Node.js. Furthermore, we could consume pre-trained tensorflow weights in Java. Here, we can apply training step as well. In this post, I created a complete beginner’s guide to TensorFlow.js.

Client side application
In this case, we can just run the code. No prerequisite installation is required. I will create a hello.html file and reference tensorflow js library in head tag. This reference offers to find tensorflow related objects under tf variable. There might be up-to-date version of the library. You should check it in the official site.
🙋♂️ You may consider to enroll my top-rated machine learning course on Udemy
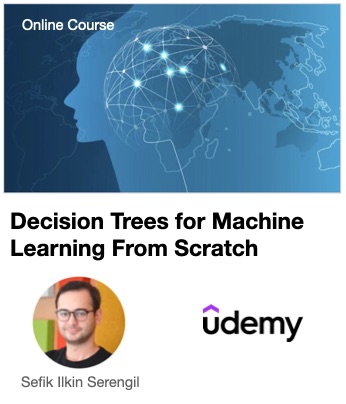
Also, I need to define an another script tag after tensorflow js referencing. I have to construct neural networks here.
<html> <head> <script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@0.12.5"> </script> <!-- Place your code in the script tag below --> <script> </script> </head> <body> </body> </html>
Problem
I will construct a model for XOR problem. Let’s create the data set first. Here, xtrain stores all potential inputs whereas ytrain stores xor logic gate results respectively as one-hot encoded. I mean that [1, 0] refers to firing 0 whereas [0, 1] refers to firing 1 as xor result.
const xtrain = tf.tensor2d([[0, 0], [0, 1], [1, 0], [1, 1]]); const ytrain = tf.tensor2d([[1, 0], [0, 1], [0, 1], [1, 0]]);
Constructing model
We can construct a neural networks model. I will create a sequential model. Input layer consists of 2 nodes because there 2 input features in xor data set. First and single hidden layer will have 5 nodes and its activation function will be sigmoid. Finally, output layer will have 2 nodes because xor data set has 2 output classes. Activation function of output layer should be softmax because this is a classification problem.
const model = tf.sequential(); model.add(tf.layers.dense({units: 5, activation: 'sigmoid', inputShape: [2]})); model.add(tf.layers.dense({ units: 2, activation: 'softmax' }));
Learning objective
Now, we can specify the optimization algorithm and loss function to train the model. You have to use categorical crossentropy loss function if you use softmax activation function in the output layer. Moreover, I would like to train the model with Adam optimization algorithm to be learnt faster.
var learning_rate = 0.1 model.compile({loss: 'categoricalCrossentropy', optimizer: tf.train.adam(learning_rate)});
Training
Time to train the network. You might remember that we run fitting and prediction respectively in python. Here, running is a little different. Fit command is handled asynchronously. That’s why, you must not run fit and predict commands in separate lines as demonstrated below. Otherwise, predict command dumps the results before training.
//you should not run the prediction in this way const history = model.fit(xtrain, ytrain, {epochs: 200}) console.log("fit is over") model.predict(xtrain).print();
Fit command should include prediction as illustrated below.
const history = model.fit(xtrain, ytrain, {epochs: 200}) .then(()=>{ console.log("fit is over") //model.predict(tf.tensor2d([[0, 0], [0, 1], [1, 0], [1, 1]])).print(); model.predict(xtrain).print(); });
Testing in the browser
Coding is over for client side solution. Now, you can open the hello.html file in the browser. Do not surprise when you see the blank page. You can see the final predictions by pressing F12 button in chrome. Or you can access the same place under Settings (3 points on the right top side) > More tools > Developer tools > Console tab.

So, we can successfully create the Machine Learning in the browser as shown above. But this is too beyond the ML in browser. Let’s see how.
Server side application
Server side capabilities enabled for javascript in Node.js recently. We can run the (almost) same code in Node.js server. In this case, you have to install Node.js into your computer. I installed the recommended version 8.11.4 for today. You can run node command in the command prompt after installation.
Installing TensorFlow.js for Node.js
You should run the following command if you run the node.js first time. This creates packages.json file in the current directory. Otherwise, tensorflow.js installation would not complete successfully. BTW, I run the command on my desktop.
npm init
You can install TensorFlow.js package after initialization. (double dash and save. it seems like single dash in the browser)
npm install @tensorflow/tfjs –save
Coding
That’s it! Your environment is ready. Please create a hello.js file. Content of the file will be look like this.
var tf = require('@tensorflow/tfjs'); const model = tf.sequential(); model.add(tf.layers.dense({units: 5, activation: 'sigmoid', inputShape: [2]})); model.add(tf.layers.dense({ units: 2, activation: 'softmax' , outputShape: [2] })); model.compile({loss: 'categoricalCrossentropy', optimizer: tf.train.adam(0.1)}); const xtrain = tf.tensor2d([[0, 0], [0, 1], [1, 0], [1, 1]]); const ytrain = tf.tensor2d([[1,0],[0,1],[0,1],[1,0]]); const history = model.fit(xtrain, ytrain, {epochs: 200}) .then(()=>{ console.log("fit is over") //model.predict(tf.tensor2d([[0, 0], [0, 1], [1, 0], [1, 1]])).print(); model.predict(xtrain ).print(); });
As seen, we’ve run the same code. Model has learnt the principles of xor logic gate successfully.

So, we have mentioned the javascript version of TensorFlow in this post. TensorFlow is not just a tool for research. For instance, Facebook developed both PyTorch and Caffe2 frameworks for deep learning. However, Facebook uses PyTorch as research purposes whereas it uses Caffe2 for production. On the other hand, Google enabled TensorFlow for both research and production. It seems that we will see TensorFlow.js much more common in the following days.
Like this blog? Support me on Patreon